Electron – チュートリアルその2 Typescript を使用する
- 2024.01.20
- Electron
- Electron, Javascript
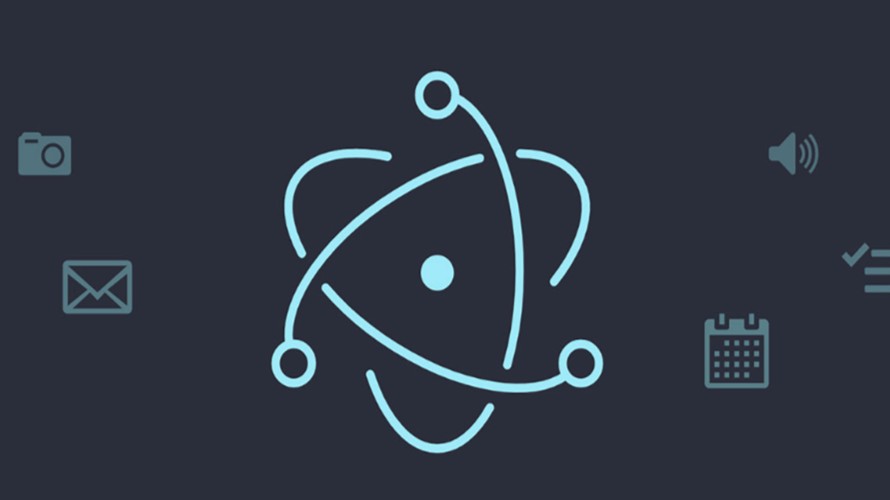
目次
概要
Electron – チュートリアルその1 プロジェクトの作成では Javascript で Electron のコードを作成しましたが、今回は Electron のプロジェクトで Typescript を使用する方法について紹介します。
Advertisement
手順
コード
コード全体は以下を参照ください。
electron-examples/02_electron-tutorial-use-typescript
1. パッケージを作成する
npm init
コマンドで Node パッケージを作成します。
npm init
package name: (electron-sample) electron-example
version: (1.0.0) 1.0.0
description: Electron example
entry point: (index.js) main.ts
test command:
git repository:
keywords:
author: papillon
license: (ISC) MIT
現時点で、ディレクトリ構成は以下のようになっています。
electron-example
├── node_modules
├── package-lock.json
└── package.json
2. Electron、Typescript をインストールする
Electron 及び Typescript をインストールします。
npm install -D electron typescript
3. 必要なファイルを作成する
コンパイル設定ファイル tsconfig.json
を作成します。
"target": "es2016"
: コンパイル後のコードの ECMAScript のバージョン"module": "commonjs"
: Common JS モジュールとして出力する"strict": true
: 型チェックなどのバリデーションを厳しくする"outDir": "./build"
:tsconfig.json
があるディレクトリを起点に./build
にコンパイルしたファイルを出力する
tsconfig.json
{
"compilerOptions": {
"target": "es2016",
"module": "commonjs",
"strict": true,
"outDir": "./build"
}
}
エントリポイントとなる main.ts
を作成します。
main.ts
import { app, BrowserWindow } from "electron";
const createWindow = () => {
const win = new BrowserWindow();
win.loadFile("index.html");
};
app.whenReady().then(createWindow);
また、ウィンドウに表示する index.html
を作成します。
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
</head>
<body>
<h1>Hello World</h1>
</body>
</html>
現時点で、ディレクトリ構成は以下のようになっています。
electron-example
├── index.html
├── main.ts
├── package-lock.json
├── package.json
└── tsconfig.json
4. package.json のコマンドを変更する
コンパイルは tsc
コマンドで行います。npx tsc -p .
を実行すると、./build
ディレクトリにコンパイル後の Javascript ファイルが出力されます。
electron-example
├── build
| └── main.js
├── index.html
├── main.ts
├── package-lock.json
├── package.json
└── tsconfig.json
npm start
を実行したときに、Typescript のビルドを行い、生成された Javascript ファイルを使用して Electron を実行できるように、package.json
にコマンドを追加します。
エントリポイントは、ビルドにより生成される ./build/main.js
に変更します。
package.json
{
"name": "electron-example",
"version": "1.0.0",
"description": "Electron example",
"main": "./build/main.js",
"scripts": {
"start": "tsc -p . && electron ."
},
"author": "papillon",
"license": "MIT",
"devDependencies": {
"electron": "^28.1.3",
"typescript": "^5.3.3"
}
}
-
前の記事
Electron – チュートリアルその1 プロジェクトの作成 2024.01.20
-
次の記事
Electron – チュートリアルその3 BrowserWindow のプロパティ 2024.01.20