概要
shadcn/ui の Table コンポーネントにソート機能を実装する方法を解説します。
前提
以下のコードは React – shadcn/ui の Table コンポーネントについて解説 | pystyle で作成した動的なテーブルの実装の機能追加になります。 まず、上記のページでテーブルを実装し、そのコードに以下のコードを追加してください。
ソート機能を実装する
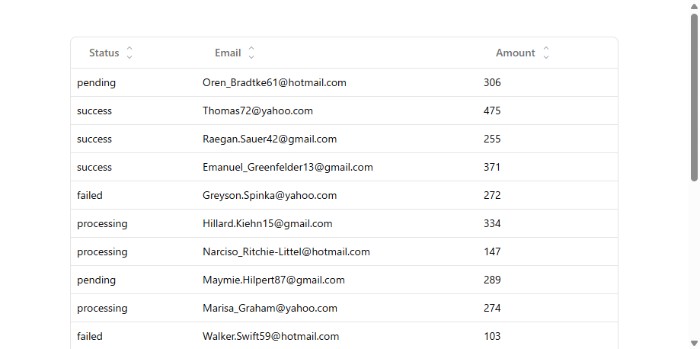
1. Button コンポーネントを追加
ページ遷移ボタンの実装には、Button コンポーネントを使用するため、インストールします。
2. ソート機能付きのヘッダコンポーネントを作成
src/payment/column-header.tsx
を作成します。
- ヘッダの項目をクリックすると、ソート状態が変更されるようにボタンにします。
- クリックした場合、
column.toggleSorting()
を実行して、ソート状態を変更します。 - 現在のソート状態がわかるように、ヘッダのテキストの右側に △▽ を追加します。
column.getIsSorted()
で現在のソート状態を取得し、昇順の場合は △、降順の場合は ▽ を強調表示します。
src/payment/column-header.tsx
3. カラム定義を変更する
src/payment/columns.tsx
4. ソート機能の追加
テーブルを実装した src/payment/payment-table.tsx
を以下のように変更します。
src/payment/payment-table.tsx
解説
詳しくは Sorting Guide | TanStack Table Docs を参照してください。
ソート状態を管理するための state を作成し、table のソート状態と連携します。
- onSortingChange: テーブルのソート状態が変更された場合に呼ばれるコールバック関数を設定します。
- state.sorting: ソート状態を管理する state を設定します。
- getSortedRowModel: ソート状態に応じた行モデルを取得する関数を設定します。
コメント