目次
概要
NumPy の三角関数、逆三角形関数、双曲線関数、逆双曲線関数について解説します。
numpy.sin
正弦関数 ($\sin(x)$) の値を計算します。
numpy.sin(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "sin">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
角度 (ラジアン) |
返り値
名前 | 説明 |
---|---|
y | 正弦関数の値。x と同じ形状の配列。x がスカラならこれはスカラです。 |
サンプルコード
In [1]:
import numpy as np
from matplotlib import pyplot as plt
x = np.linspace(0, np.pi * 2, 100)
y = np.sin(x)
fig, ax = plt.subplots(figsize=(6, 3))
ax.plot(x, y)
ax.grid()
plt.show()
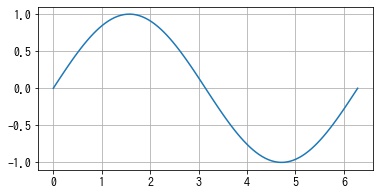
numpy.cos
余弦関数 ($\cos(x)$) の値を計算します。
numpy.cos(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "cos">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
ラジアン単位の入力配列。 |
返り値
名前 | 説明 |
---|---|
y | 余弦関数の値。x と同じ形状の配列。x がスカラならこれはスカラです。 |
サンプルコード
In [2]:
x = np.linspace(0, np.pi * 2, 100)
y = np.cos(x)
fig, ax = plt.subplots(figsize=(6, 3))
ax.plot(x, y)
ax.grid()
plt.show()
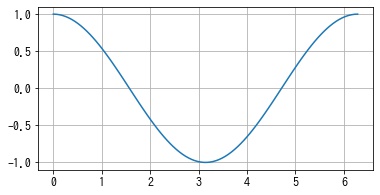
numpy.tan
正接関数 ($\tan(x)$) の値を計算します。
numpy.tan(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "tan">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
入力配列. |
返り値
名前 | 説明 |
---|---|
y | 正接関数の値。x と同じ形状の配列。x がスカラならこれはスカラです。 |
サンプルコード
In [3]:
x = np.linspace(-np.pi / 2, np.pi / 2, 100)
y = np.tan(x)
fig, ax = plt.subplots(figsize=(3, 3))
ax.plot(x, y)
ax.grid()
ax.set_ylim(-10, 10)
plt.show()
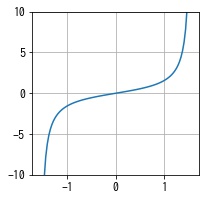
numpy.arcsin
逆正弦関数 ($\arcsin(x)$) の値を計算します。
numpy.arcsin(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "arcsin">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
単位円上の座標。実数の場合、定義域は $[-1, 1]$ です。 |
返り値
名前 | 説明 |
---|---|
angle | 逆正弦関数の値。x と同じ形状の配列。x がスカラならこれはスカラです。 |
サンプルコード
In [4]:
x = np.linspace(-1, 1, 100)
y = np.arcsin(x)
fig, ax = plt.subplots(figsize=(3, 6))
ax.plot(x, y)
ax.grid()
plt.show()
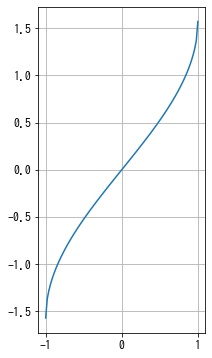
numpy.arccos
逆余弦関数 ($\arccos(x)$) の値を計算します。
numpy.arccos(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "arccos">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
単位円上の座標。実数の場合、定義域は $[-1, 1]$ です。 |
返り値
名前 | 説明 |
---|---|
angle | 逆余弦関数の値。x と同じ形状の配列。x がスカラならこれはスカラです。 |
サンプルコード
In [5]:
x = np.linspace(-1, 1, 100)
y = np.arccos(x)
fig, ax = plt.subplots(figsize=(3, 6))
ax.plot(x, y)
ax.grid()
plt.show()
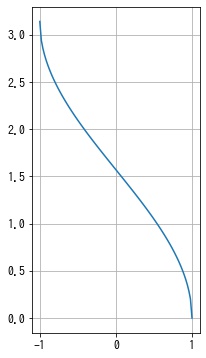
numpy.arctan
逆正接関数 ($\arctan(x)$) の値を計算します。
numpy.arctan(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "arctan">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
返り値
名前 | 説明 |
---|---|
out | 逆正接関数の値。x と同じ形状の配列。x がスカラならこれはスカラです。 |
サンプルコード
In [6]:
x = np.linspace(-3, 3, 100)
y = np.arctan(x)
fig, ax = plt.subplots(figsize=(6, 3))
ax.plot(x, y)
ax.grid()
plt.show()
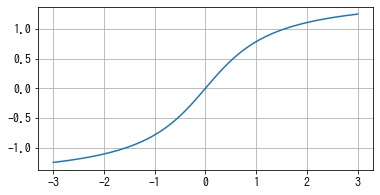
numpy.arctan2
x1/x2の要素別円弧正接を正しく選択します。
numpy.arctan2(x1, x2, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "arctan2">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x1 | array_like, real-valued | |
y 座標。 | ||
x2 | array_like, real-valued | |
x 座標。x2 は、x1 の形状と一致するようにブロードキャスト可能でなければなりません。 |
返り値
名前 | 説明 |
---|---|
angle | ラジアン単位の角度の配列で、範囲は [-pi, pi]。x1 と x2 の両方がスカラである場合、これはスカラです。 |
numpy.sinh
ハイパボリックサイン ($\sinh(x)$) の値を計算します。
numpy.sinh(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "sinh">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
入力配列。 |
返り値
名前 | 説明 |
---|---|
y | ハイパボリックサインの値。x がスカラならこれはスカラです。 |
numpy.cosh
ハイパボリックコサイン ($\cosh(x)$) の値を計算します。
numpy.cosh(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "cosh">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
入力配列。 |
返り値
名前 | 説明 |
---|---|
out | ハイパボリックコサインの値。x と同じ形状の出力配列。x がスカラならこれはスカラです。 |
numpy.tanh
ハイパボリックタンジェント ($\tanh(x)$) の値を計算します。
numpy.tanh(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "tanh">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
入力配列。 |
返り値
名前 | 説明 |
---|---|
y | ハイパボリックタンジェントの値。x がスカラならこれはスカラです。 |
numpy.arcsinh
逆ハイパボリックサイン ($\arcsinh(x)$) の値を計算します。
numpy.arcsinh(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "arcsinh">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
入力配列。 |
返り値
名前 | 説明 |
---|---|
out | 逆ハイパボリックサインの値。x と同じ形状の配列。x がスカラならこれはスカラです。 |
numpy.arccosh
逆ハイパボリックコサイン ($\arccosh(x)$) の値を計算します。
numpy.arccosh(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "arccosh">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
入力配列。 |
返り値
名前 | 説明 |
---|---|
arccosh | 逆ハイパボリックコサインの値。x と同じ形状の配列。x がスカラならこれはスカラです。 |
numpy.arctanh
逆ハイパボリックタンジェント ($\arctanh(x)$) の値を計算します。
numpy.arctanh(x, /, out=None, *, where=True, casting="same_kind", order="K", dtype=None, subok=True[, signature, extobj]) = <ufunc "arctanh">
引数
名前 | 型 | デフォルト値 |
---|---|---|
x | array_like | |
入力配列。 |
返り値
名前 | 説明 |
---|---|
out | 逆ハイパボリックタンジェントの値。x と同じ形状の配列。x がスカラならこれはスカラです。 |
コメント